Tomorrow ,28th March, sees the world-wide celebration of National Arduino Day.
It is a world-wide event where people come together to celebrate their Arduino projects. So, as promised, here’s our contribution to National Arduino Day. Steve Nice, C.E.O. of Reconnix, talks about his adventures with the Arduino. Enjoy!
Adventures with the Internet of Things Part 1 – Getting Arduino, Adafruit CC3000 and Phant Data Logging Engine Working Together.
I was interested to see how I could log data from some sensors and emulate an IoT device.
Rather than using an Ethernet shield for my Arduino I wanted to go wireless. I opted for the Adafruit CC3000 as there are excellent resources on Adafruit’s website. For logging the data I came across a free data logging engine and service from the excellent SparkFun – data.sparkfun.com. This allows you to set up a public stream for you to send your data to. They have also open sourced the software called Phant. This allows you to create your own IoT data logging service.
Adafruit CC3000
The CC3000 comes in 2 flavours – breakout board or shield. I opted for the shield version. Once I had soldered the pins to the Arduino I plugged it in and connected it up to my Linux machine.
Following instructions I downloaded the CC3000 libraries then loaded the buildtest sketch. This sketch scans for wireless networks, connects the SSID defined, requests an IP via DHCP, does a DNS name resolution for www.adafruit.com and finally pings it. If you’ve done your soldering correctly it should work. If it doesn’t work check your soldering.
Here’s the output from the buildtest sketch –
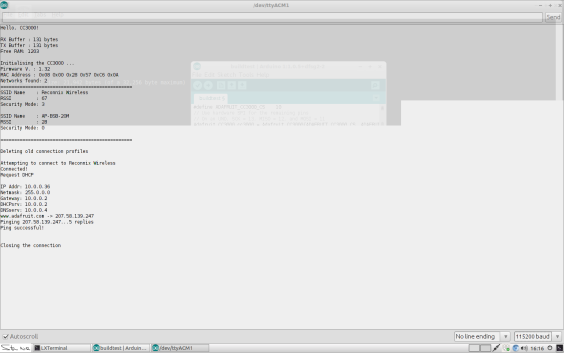
Now try the webclient sketch that comes with the CC3000 library. Remember to set the wireless details.
Phant Server – Open Source data logging engine
With the wireless working the next step was to install the data logging engine Phant. Using the instructions at https://github.com/sparkfun/phant I opted for the Nodejs package. It was straightforward and only took a few minutes to install. Connect to port 8080 and you can see the options to create, deploy or explore. Click create to create a new stream and follow the instructions.
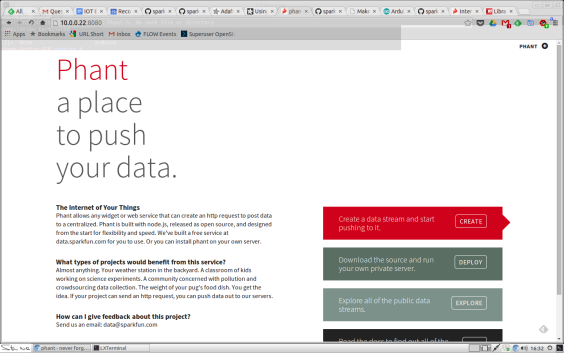
Keep hold of the public and private key as you’ll need them for the sketch later on. Make sure your stream is working by putting a test string in your browser –
https://IP:8080/input/public_key?private_key=XXXXXXXXXXXXXx&val1=33.4
(Replace public_key with your streams public key, XXXXXXXXXXXXX with your private key and val1 with your Field name(s)) For example –
https://data.sparkfun.com/input/Jxyjr7DmxwTD5dG1D1Kv?private_key=gzgnB4VazkIg7GN1g1qA&brewTemp=33.4
You should see a success message. Go to the EXPLORE link from the start page and you should see the data that you’ve just posted. If not check that you’ve entered the correct keys and field values.
Phant Arduino
Now we have to combine a Phant Arduino sketch with the CC3000 sketch. Firstly load the Phant Ardunio library as described here.
Load up the webclient sketch that you used earlier to test the CC3000. We need to add and remove a few lines. Firstly add the Phant headers –
#include <Phant.h>
Define your wireless details
#define WLAN_SSID "Wireless name" // cannot be longer than 32 characters!
#define WLAN_PASS "Wireless password"
Define your Phant keys from the stream you created earlier.
// Phant keys etc
Phant phant("10.0.0.22", "Public_key", "Private_key");
Tell sketch the IP of your Phant server (yes the IP is separated by commas)
//IP of phant server
uint32_t ip = cc3000.IP2U32(10,0,0,22);
Main code. This is the part that uses the Phant.add and post functions. You must add your values using the Phant.add function then post them immediately with the Phant.post function.
Adafruit_CC3000_Client www = cc3000.connectTCP(ip, 8080);
if (www.connected()) {
phant.add("test",20);
phant.add("test",30);
// etc etc etc
www.println(phant.post());
phant.add("test",20);
phant.add("test",30);
// etc etc etc
www.println(phant.post());
All being well you should see the stream updated with the values posted.
What next? Attached the CC3000 to a breadboard along with some sensors and stream some live data.

Here’s the complete sketch –
#include
#include
#include
#include
#include
#include "utility/debug.h"
#define ADAFRUIT_CC3000_IRQ 3
#define ADAFRUIT_CC3000_VBAT 5
#define ADAFRUIT_CC3000_CS 10
Adafruit_CC3000 cc3000 = Adafruit_CC3000(ADAFRUIT_CC3000_CS, ADAFRUIT_CC3000_IRQ, ADAFRUIT_CC3000_VBAT, SPI_CLOCK_DIVIDER);
#define WLAN_SSID "Wireless name" // cannot be longer than 32 characters!
#define WLAN_PASS "Wireless password"
#define WLAN_SECURITY WLAN_SEC_WPA2
#define IDLE_TIMEOUT_MS 3000
// Phant keys etc
Phant phant("10.0.0.22", "Public_key", "Private_key");
//IP of phant server
uint32_t ip = cc3000.IP2U32(10,0,0,22);
void setup(void)
{
Serial.begin(9600);
Serial.println(F("Hello, CC3000!\n"));
Serial.print("Free RAM: "); Serial.println(getFreeRam(), DEC);
/* Initialise the module */
Serial.println(F("\nInitializing..."));
if (!cc3000.begin())
{
Serial.println(F("Couldn't begin()! Check your wiring?"));
while(1);
}
Serial.print(F("\nAttempting to connect to ")); Serial.println(WLAN_SSID);
if (!cc3000.connectToAP(WLAN_SSID, WLAN_PASS, WLAN_SECURITY)) {
Serial.println(F("Failed!"));
while(1);
}
Serial.println(F("Connected!"));
/* Wait for DHCP to complete */
Serial.println(F("Request DHCP"));
while (!cc3000.checkDHCP())
{
delay(100); // ToDo: Insert a DHCP timeout!
}
/* Display the IP address DNS, Gateway, etc. */
// while (! displayConnectionDetails())
{
delay(1000);
}
Adafruit_CC3000_Client www = cc3000.connectTCP(ip, 8080);
if (www.connected()) {
phant.add("test",20);
// ADD MORE PHANT.ADD here
www.println(phant.post());
Serial.println(F("Finished"));
} else {
Serial.println(F("Connection failed"));
return;
}
Serial.println(F("-------------------------------------"));
/* Read data until either the connection is closed, or the idle timeout is reached. */
unsigned long lastRead = millis();
while (www.connected() && (millis() - lastRead < IDLE_TIMEOUT_MS))
{
while (www.available())
{
char c = www.read();
Serial.print(c);
lastRead = millis();
}
}
www.close();
Serial.println(F("-------------------------------------"));
/* You need to make sure to clean up after yourself or the CC3000 can freak out */
/* the next time your try to connect ... */
Serial.println(F("\n\nDisconnecting"));
cc3000.disconnect();
}
void loop(void)
{
delay(1000);
}
Pingback: Reconnix The R.I.S.E. of the Arduino.()